목록Python Study (25)
Studio KimHippo :D
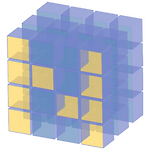
bool_ : True or False int_ : 기본 정수 타입 (C에서의 long) intc : C에서의 int와 동일 intp : 인덱싱에서 사용하는 정수 int8 : -128 ~ 127 int16 : -32768 ~ 32767 int32 : -2147483648 ~ 2147483647 int64 : -9223372036854775808 ~ -9223372036854775807 uint8 : 부호없는 정수 (0 ~ 255) uint16 : 부호없는 정수 (0 ~ 65535) uint32 : 부호없는 정수 (0 ~ 4294967295) uint64 : 부호없는 정수 (0 ~ 18446744073709551615) float_ : float64의 약칭 flot16 : 반정밀 부동 소수점 (부호비트, ..
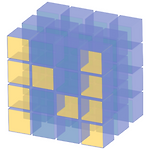
# -*- coding : utf-8 -*- import numpy as np # NOTE : NumPy의 구조화된 배열 name = ['Alice', 'Bob', 'Cathy', 'Doug'] age = [25, 45, 37, 19] weight = [55.0, 85.5, 68.0, 61.5] # NOTE : 빈 컨테이너 배열 생성 data = np.zeros(4, dtype = {'names' : ('name', 'age', 'weight'), 'formats' : ('U10', 'i4', 'f8')}) data['name'] = name data['age'] = age data['weight'] = weight data Out [1] : array([('Alice', 25, 55. ), ('Bob'..
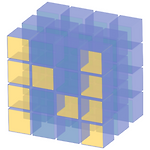
# -*- coding : utf-8 -*- import numpy as np # NOTE: 팬시 인덱싱 # 팬시 인덱싱 : 단일 스칼라 대신 인덱스 배열을 반환 # 이로써 복잡한 배열 값의 하위 집합에 매우 빠르게 접근하여 수정가능 rand = np.random.RandomState(42) x = rand.randint(100, size = 10) x # NOTE : 세 개의 다른 요소에 접근하고자 할 때 print([x[3], x[7], x[2]]) # NOTE : 인덱스의 단일 리스트나 배열을 전달 ind = [3, 7, 4] print(x[ind]) # NOTE : 결과의 형상이 대상 배열의 형상이 아니라 인덱스 배열의 형상을 반영 ind = np.array([[3, 7], [4, 5]]) pri..
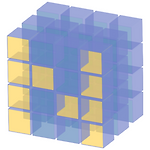
# -*- coding : utf-8 -*- %matplotlib inline import matplotlib.pyplot as plt import numpy as np # NOTE # 벡터화하는 연산을 위해서는 NumPy의 ufuncs말고 브로드캐스팅 기능이 있다. # 브로드캐스팅은 다른 크기의 배열에 이항 ufuncs를 적용하기 위한 규칙이다. a = np.array([0, 1, 2]) b = np.array([5, 5, 5]) print(a + b) print(a + 5) M = np.ones((3,3)) M + a Out [1] : [5 6 7] [5 6 7] Out [2] : array([[1., 2., 3.], [1., 2., 3.], [1., 2., 3.]]) a2 = np.arange(3)..
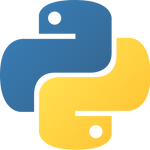
리스트 자료형 # -*- coding : utf-8 -*- a = [] b = [1, 2, 3] c = ['Life', 'is', 'too', 'short'] d = [1, 2, ['Life', 'is']] # NOTE : 리스트 인덱싱 x = [1, 2, 3] print(x) print(x[0]) print(x[0] + x[2]) print(x[-1]) # NOTE : 리스트 슬라이싱 y = [1, 2, 3, 4, 5] print(y[0:4]) print(y[:3]) # !CAUTION! : 리스트 슬라이싱 할 때 a[x:y]라 하면, # x부터 y까지가 아니라, x부터 y-1까지다. print(y[2:]) # NOTE : 리스트 연산 print(x+y) print(x*3) print(len(x)) z..
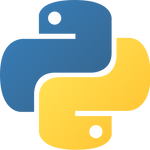
문자열 포매팅 # -*- coding : utf-8 -*- print('I eat %s apples.' % 'five') print('I eat %d apples.' % 3) number = 7 day = 'three' print('I eat %d apples.' % number) print('I ate %d apples. so I was sick for %s days.' % (number, day)) print('Error is %d%%.' % 98) # NOTE : 오른쪽 정렬 print('%10s' % 'hi') # NOTE : 왼쪽 정렬 print('%-10s' % 'hi') print('%s Jane' % 'hi') print('%-10s Jane' % 'hi') # NOTE : 소수점 표시 p..
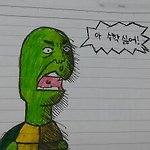
# -*- coding: utf-8 -*- # NOTE : 삽입 정렬 def insertion_sort(): arr = list(map(int, input('자료를 입력해 주세요. : ').split(' '))) for o_rep in range(1, len(arr)): for i_rep in range(o_rep): if arr[o_rep] == arr[i_rep]: pass else: if arr[o_rep] < arr[i_rep]: arr[o_rep], arr[i_rep] = arr[i_rep], arr[o_rep] print(arr) return arr print(insertion_sort())
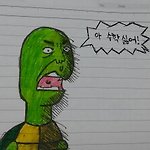
# -*- coding : utf-8 -*- # NOTE : KDA를 입력받아 mvp인 플레이어 추출 player_name = ['a1', 'a2', 'a3', 'a4', 'a5', 'b1', 'b2', 'b3', 'b4', 'b5' ] player_score = {} for rep in player_name: kda = list(map(int, input('당신의 KDA를 입력하십시오. : ').split(' '))) score = (kda[0] * 2 + kda[2]) / kda[1] player_score[rep] = score for rep in range(len(player_score)): if list(player_score.values())[rep] == max(player_score.val..